Exercise 3.4
- Evaluate each expression assuming that the following declarations
have been made.
- Simplify as much as possible.


- Write a boolean expression that will be true if and only if the int
variable i satisfies the condition 0
i 5.
- Use de Morgan's laws and the fact that !!p is equivalent to p to
simplify each expression.

- In addition to de Morgan's laws, there are a number of other laws
that can be used to simplify boolean expressions. A useful list of
equivalences is given in the following table. (The symbol
is used
to mean that two boolean expressions are equivalent.)
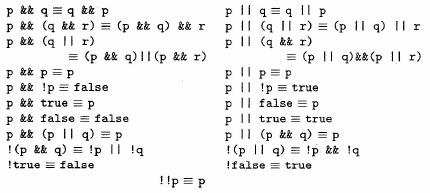
Use these relationships to simplify each of the following expressions
as much as possible.
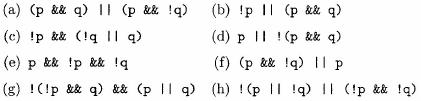
- The exclusive or operator, which is sometimes written as
,has the
following truth table.
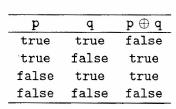
Write a Java expression that is true if and only if p q is true.
| |