Exercise 2.1
- Evaluate each valid expression and state the type of the result. If the expression
is invalid, give the reason.
- 2 + 7 / 2
- 55 / 5
- ( 8 - 3 * 2) * 4 - 1
- 16 - 24 / 6 / 2
- 5(6 + 3)
|
- 20 / (8 - 2)
- 2E+02 + 02
- 20 * .4
- 4 - [6 - (2 - 7)]
- 4e-3 + 2e-1
|
- Evaluate each valid expression. If the expression is invalid, give the reason.
- 39 div 3
- 39 mod 3
- 24 div -5
- 27 mod 4
- 15 div 24
- 24.5 div 3
- -15 div 7
|
- -4 div 9
- -56 div -11
- -86 div 20
- 46 div 9 div 2
- 23 mod 13 mod 3
- 37 div 8 mod 2
- 5 div (8 div 10)
|
- Evaluate.
- 5 * (20 div 5)
- 48 div 6 * 6
- (65 div 10) * 10
- 6 * (28 div 6)
- 3 * (20 div 3) + 20 mod 3
- 8 * (60 div 8) + 60 mod 8
- 17 * (99 div 17) + 99 mod 177
- 7 *(-24 div 7) + (-24) mod 7
- -20 div 9 * 9 - 20 mod 9
- (-13 div 3) * 3 + (-13 mod 3)
- Assuming that the following assignments have been made:
var m : int := 30 var n : int := 40
var x : real := 1.5 var y : real := 4.0
evaluate each expression, writing real valued answers correct to four decimal places
- 5 * (x - (-2) div 7) + n div m mod 4
- m * (n + 28 div (n div 12)) - x
- (x + 5) * ((-y / 8e0 + 7e1) / 2e1 + 1)
- (m - 10) / (n + 10) * (x + y) / (x - y)
- Write as a Turing expression, using the fewest brackets possible. (Assume that x and y are real.)
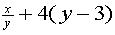
- x3 + y3

- Evaluate each valid expression. If the expression is invalid, give the reason.
- 2 ** 8
- 10.0 ** - 5
- 8 ** (1 / 3)
|
- 16 ** (- 1 / 2)
- -25 ** 0.5
- (-36) ** 0.5
|
|
|