Exercise 7.5
- The following fragment determines the largest number in list, an
array of double values. Rewrite the fragment to make it clearer.
double largest = list [0] ;
int i = 1;
while (i < list.length)
if (list[i++] > largest)
largest = list[i-1];
- Suppose that you are required to read a set of values and determine
the given quantity. For which ones (if any) would you need to use an
array?
(a) the largest value | (b) the median |
(c) the mean | (d) the range |
- Identify and correct the error in each declaration.
double a = new double[10];
-
int[] b = new int[];
-
char[][] c = char[30][];
float [][] d = new float[][10] ;
-
int[] e = new {3,5,2,9,1};
- A programmer, using a square two-dimensional array of int values
called table, wanted to sum the elements along the main diagonal
(the diagonal whose elements are table[0][0], table[1][1], and
so on). To do this the programmer wrote
int total = 0;
for (int i = 0; i < table.length; i++)
for (int j = 0; j < table.length; j++)
total += table[i] [j];
- What does the fragment actually do?
- Write a fragment that does set total to the sum of the elements
of the main diagonal.
- Write a fragment that sets total to the sum of the elements of
the other diagonal of table.
- Does the statement double[] list; create an array of double elements?
Explain.
- State the value of the indicated element after execution of the given
declaration.
(a) a[4] after int[] a = new int [20];
(b) b[23] after boolean[] b = new boolean [50];
(c) c[2] after int[] c = {4,7,2,8};
(d) d[33] after double[][] d = new double[100][];
- How many elements can be stored in the arrays created by the following declarations?
(a) double[] a = new double [10] ;
(b) byte[] b = new byte [30] ;
(c) int [][] c = new int [10][20] ;
(d) char[][][] d = new char[5][4][50] ;
- Given that list is a one-dimensional array of int values, write fragments
to print each value.
(a) the number of occurrences of the value zero,
(b) the product of all the elements,
(c) the sum of the positive elements,
(d) the minimum value.
- Given that table is a two-dimensional rectangular array, write fragments
to print each value.
(a) the number of elements
(b) the sum of the elements,
(c) the number of values that are multiples of three,
(d) the positive difference between the largest and smallest values
in the array.
- A polynomial in x of degree n is an expression of the form

The values a0, a1, ... ,an are called the coefficients of the polynomial.
Complete the definition of the method eval so that it returns the
value at x of a polynomial whose coefficients are stored in the array a.
public static double eval (double[] a, double x)
- Complete the definition of the method randomize whose header is
public static int[] randomize (int n)
The method should return an array of size n whose elements are the
values 0 ... n - 1 ordered randomly. As an example, randomize(5)
might return [4, 2, 0, 3, 1].
- Lacsap's Triangle is a triangular array of numbers in which each row
starts and ends with the row number and each interior value is the
sum of the two values on either side of it in the preceding row. The
following diagram shows the first five rows of Lacsap's Triangle.
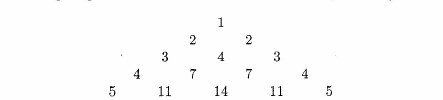
Write a program that asks the user for a positive integer and, once
a satisfactory value has been supplied, produces that many rows of
Lacsap's Triangle. For simplicity, do not try to print the triangle in
the symmetrical form shown here.
| |