3.3 The if Statement
In performing a task, we often want to take one of two possible actions,
depending on the conditions that exist at the time. For example
if you have homework, then
you should do it,
otherwise
you can go to a movie.
To accommodate this type of situation, Processing uses an if statement
having a form similar to the conditional English statement shown above.
if (<boolean expression>)
<statement1>
else
<statement2>
The if statement’s action is controlled by an expression that must
be of type boolean. If <boolean expression> is true, <statement1> is
executed; if <boolean expression> is false, <statement2> is executed. In
either case, only one of the statements is performed.
Notice the parentheses that surround the <boolean expression>. These
parentheses are not optional. Without them, the syntax of the statement
is incorrect.
Notice also the way in which indentation is used in the if statement.
Each of the alternatives is indented slightly past the first line. As we
have said before, indentation is not required by the computer but, as the
structure of programs becomes more complex, indentation becomes more
and more important if we want to make the structure of our programs clear
to a human reader.
Example 1This program compares two integers supplied by the user and prints an
appropriate message.
void setup()
{
int first = getInt("Please give one integer");
int second = getInt("and a second");
if (first == second)
println("The values are equal");
else
println("The values are not equal");
}
If a user supplies two equal values to the program, the output will be
If the user supplies unequal values, the output will be
|
|
Sometimes a special action is necessary only if a certain condition is
true while no action is necessary if the condition is false. To accommodate
this type of situation, Processing allows us to write an if statement that has no
else clause, as follows:
if (<boolean expression>)
<statement>
Here, <boolean expression> will be executed if and only if <boolean expression>
is true. If <boolean expression> is false, the program simply proceeds to
execute the next statement in the program.
Example 2The following statement warns a user if a value of mark indicates a failure
on a test.
if (mark < PASSING_MARK)
println("Mark indicates a failure");
If the value of mark is not less than the mark required to pass, no message
will be printed.
|
|
If you always prefer to have else clauses in if statements, you can
still obtain the effect of an if statement without an else clause by using an
empty statement. An empty statement, as its name implies, has nothing in
it (except for its terminating semi-colon). Despite its strange appearance
(or lack of appearance), an empty statement can be used wherever any
other type of statement can be used.
Example 3Each of the following statements has the same effect: the value of x will be
printed if and only if x > 0.
(a) if (x > 0)
println("x = " + x);
(b) if (x > 0)
println("x = " + x);
else
;
(c) if (x <= 0)
;
else
println("x = " + x);
|
|
Frequently, when using if statements, we want to perform actions
that require more than a single statement. To do this, we can create a
compound statement or block, a group of zero or more statements enclosed
by brace brackets.
Example 4The following fragment checks on the sign of a value. If it is negative, the
fragment prints a warning message and makes the value positive.
if (value < 0)
{
println("Negative value set to positive");
value = abs(value);
}
|
|
Exercise 3.3
- Rewrite the following program using the indentation style shown
in the text.
void setup(){final int VOTING_AGE=18;int age;boolean eligible;
age=getInt("");if(age>=VOTING_AGE)eligible=true;
else eligible=false;if(eligible)println(
"Eligible to vote");else println(
"Not eligible to vote");}
- What would the program print if it were given input of 19?
- Replace the first if statement with a single assignment to the
boolean variable eligible.
- Write Processing statements to perform each task.
- Add one to the value of zeroCount if the variable total has the
value zero.
- Write an appropriate message depending on whether or not the
value of the int variable n is a perfect square.
- Add one to the value of pageCount if the variable lineCount is
greater than pageLength.
- Set the value of the boolean variable leftSide to true if the
int variable page is even, and to false if page is odd.
- Write the simplest statement that has the same effect as the given
statement.
if (x != y)
;
else
println("Values are equal");
if (answer <= maxValue)
;
else
println("Answer is wrong");
- Write a program that prompts the user for two integers, determines
whether or not the first number is a multiple of the second, and then
prints both numbers and an appropriate message.
- Write a program that reads a double value and prints both the
number and its absolute value without using the built-in method
abs method. The definition of the absolute value of x, written as |x|, is
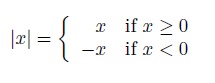
- Write a program that solves an equation of the form ax+b = 0 by first
prompting the user for the values of a and b, then solving the equation
and printing the results. The program should take appropriate action
if a is zero.
| |