1.3 Identifiers and Variables
If we are to create programs that do something other than write messages, we will need some way of storing and retrieving
values. The memory cells used to store values that can change during the execution of a program are known as variables. In order to manipulate values in Processing, we create identifier names (or, more simply, identifiers) for our variables, the methods that contain them, and the classes that contain the methods. The rules for creating any type of identifier are:
- Any character used in an identifier must be a letter of the alphabet (either upper case or lower case, in any alphabet supported by Unicode)4, a digit (0,1, ... ,9), the underscore character, or a Unicode currency symbol such as $,£ , or ¥ . Processing distinguishes between upper case and lower case letters so that, for example, x and X are recognized as different characters.
- The first character cannot be a digit.
- An identifier that you create must not be one of the following reserved words, each of which has a pre-defined and unalterable meaning in Processing.
abstract assert boolean break byte
case catch char class const
continue default do double else
extends false final finally float
for goto if implements import
instanceof int interface long native
new null package private protected
public return short static strictfp
super switch synchronized this throw
throws transient true try void
volatile while
In addition to these rules that must be followed in creating identifiers, there are also a number of conventions that should be used.
- Currency symbols (such as dollar signs) in identifiers are used by system programs and should be avoided by users.
- Long variable or method identifiers are written primarily using lower case letters with either upper case letters or underscores used to make the meaning clearer. For example, a variable to represent a starting time could be timeAtStart or time_at_start. In this text, we will use the combination of upper case and lower case letters, not the underscore character.
- Class identifiers start with an upper case letter; variable and method identifiers do not. Other characters in class identifiers follow the convention described for variables.
Example 1 From the following list, select (with reasons) those names that should not be used as identifiers of Processing variables.
firstChoice this second-time
3rdTime monthly_total Last
valueln$ x27 word Length Names that should not be used as variable identifiers are: this - a reserved word
second-time - contains a hyphen
3rdTime - starts with a digit
Last - valid but, by convention, variable identifiers start with a lower case character
valueln$ - valid but, by convention, currency symbols are avoided
word Length - contains a blank | |
It is good programming practice to use meaningful identifiers in your programs. Although it takes a bit more work to type a name like highMark than it does to type hm,the former gives the reader a much clearer idea of the use to which the identifier is to be put. In large programs, it is not unusual to have hundreds of different identifiers and, in these programs, meaningful names are almost absolutely essential for reliability. Although you will not likely be writing such large programs for some time, descriptive names will improve the clarity of any program and should be used at all times.
To reserve space in memory for variables, we must write a declaration in which we specify the type of a variable and its identifier. A simple declaration has the form
[type] [identifier];
Note that the declaration, like any statement in Processing, is terminated by a semi-colon.
Example 2 To request that space in memory be allocated to an int variable to be called age, we could write int age; We can illustrate the result of this declaration as follows:  The box represents the space in memory allocated to the variable age. Notice that, at this point, there is nothing in the box. This is because, at this point, the variable age does not yet have a value. | |
It is not necessary to use a separate declaration for each variable that we want to create. We can request that space be allocated to many variables of the same type in a single declaration using a statement of the form
[type][identifier1], [identifier2], ... , [identifierk];
Example 3 The declaration double length, width, height; creates three variables: length, width, and height, all of type double. The result of this declaration is shown in the following diagram. 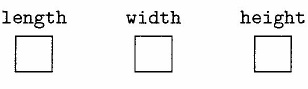 | |
When a variable is declared in a method, the declaration can appear at the start of the method (just after the opening brace bracket) or it can appear later. A variable must, however, be declared before it is first used. The practice that we follow in this text is (usually) to declare a variable just before we are about to use it.
Exercise 1.3 - What is the difference between identifiers used for classes and those used for variables and methods?
- Identify, with reasons, any identifiers that should not be used for Processing variables.
(a) digitSum (b) switch
(c) retail price (d) heightPlusDepth
(e) value@Start (f) priceIn£
(g) number-of-wins (h) ageDuGarçon
(i) average_age (j) This - Rewrite each fragment using the minimum number of declarations.
(a) int quantity; double price; int size;(b) double income;
char taxCode;
double rate;
double discount; - A program is to be written that records the results of a coin-tossing experiment. The program will need variables to record the number of heads, the number of tails, the percentage of heads (correct to four significant digits), and the experimenter's first and last initials. Write declarations to reserve space in memory of the appropriate type for each of these variables. Be sure to use identifiers that show clearly the purpose of each variable.
| |
4This includes accented Latin letters such as , , and . It also includes Greek, Cyrillic and other alphabets. Of course, your system might not be able to print such letters but they are valid in Processing.