9.1 String Objects
As we have noted previously, strings in Java are objects - instances of the
class String (in the package java.lang). A variable declared to be of type
String is a reference to a String object. We can create and initialize a
string using a constructor, just as we have done for other objects.
Example 1
The statement
String friend = new String("Kate");
creates a new string as shown in the next diagram.
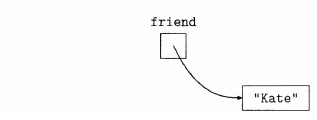
This constructor is rarely used; Java has an equivalent, simpler form that
can be used to create and initialize a variable of type String. The statement
String friend = "Kate";
has exactly the same effect as the preceding statement and is easier to
write.
|
|
It is appropriate to use a constructor explicitly in cases where we want
to produce a String object with the same value as a previously defined
String object.
Example 2
The statements
String s1 = "Sample";
String s2 = new String(s1);
would create two String objects, both containing the value"Sample".
|
|
It is possible for a variable of type String to be in a state in which it
does not refer to a string containing characters. This can occur in a number
of ways and it is important to be clear about the differences among them.
One way that this can happen arises if we write
String s1;
The result of this is an uninitialized string. An attempt to use s1 in this
state will result in an error during compilation of the program. To avoid
such compilation errors, we can initialize a string variable so that it does
not refer to a string (but is still defined) by assigning it the value null. If
we write
String s2 = null;
then we can print the string (producing the output "null") or compare
the string to other strings or compare the string to the value null. As a
third possibility, a string can be assigned the value of what is sometimes
called the empty string, a string containing no characters. We can do this
by writing
String s3 = "";
This creates a string object whose length is zero. If we print the value of
s3, it produces no output. The variables s1, s2, and s3 are illustrated in
the next diagram. As we have done with other reference variables, we have
used a dot inside s1 to indicate that it has no value and the ground symbol
with s2 to denote a null reference.
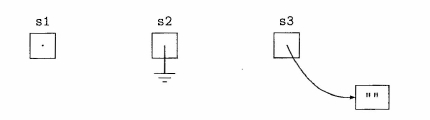
The class String contains no mutator methods; once we create a
string, its value cannot be changed. Because of this, we say that strings
are immutable objects.(There is a class StringBuffer in the java.lang package that does contain mutator
methods but we will not be using this class.) This does not mean that we cannot change values
of string variables.
Example 3
The fragment
String s = "Hello";
s = "Bonjour";
first sets the variable s to refer to a string object containing "Hello" and
then sets s to refer to a new string object containing "Bonjour" (at which
point the object containing "Hello" is lost). The result is illustrated in
the next diagram.
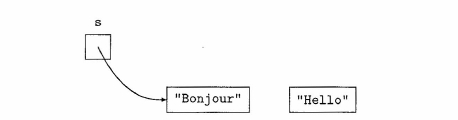
Although the string containing "Hello" still exists, it is no longer accessible.
|
|
Exercise 9.1
- What would be produced by a program containing the following fragment?
String s;
System.out.println(s);
- What would be printed by this fragment?
String s, t, u;
s = null;
t = "";
u = " ";
System.out.println("s is |" + s + "|");
System.out.println ("t is |" + t + "|");
System.out.println("u is |" + u + "|");
- What would be printed by each fragment?
(a) String s = "Sample";
String t = s;
s = "Simple";
System.out.println("s is: " + s) :
System.out.println("t is: " + t);
(b) String s = "First";
String t = new String(s);
s = "Second";
System.out.println("s is: " + s);
System.out.println("t is: " + t);
- (a) What do we mean when we say that strings are immutable?
(b) Is the following fragment legal? Explain.
String s = "old";
s = "new";
| |