Exercise 6.5
- If p and q are both variables of type Fraction, under what circumstances
will the expression p == q have the value true?
- Suppose that p and q are both of type Fraction with p representing
2/3 and q representing 1/6.
- Draw a diagram like those shown in the text to illustrate this
situation.
- If the statement p = q; is executed, draw a diagram to illustrate
the result.
- The diagram shows a Circle object of the type that we have been
using in exercises throughout this chapter.
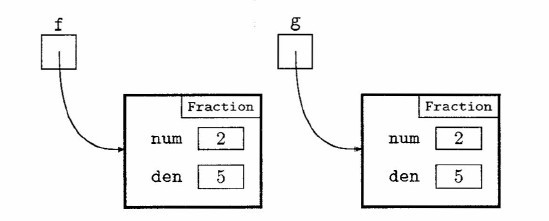
- Write a fragment to create a new reference, c2, to the same
object.
- Write a fragment to create a new Circle object c3, with the
same centre and radius as c1.
- Draw diagrams to illustrate the results of executing the code in
parts (a) and (b).
- What is the value of the expression cl == c2?
- What is the value of the expression c1 == c3?
- Write a boolean instance method called equals that returns
true if and only if one Circle object is equal to another one.
- Write a toString method for the Circle class. For a Circle
object with x = 3, Y = -4, and r = 2, the toString method
should return a String with the value:
"centre: (3,-4) radius: 2".
- You have been hired by a wicked witch with a strong interest in
children. The program that she uses to help her maintain records
has a class Child that contains the fields
private int height; // height in cm
private double mass; // mass in kg
The witch wants you to write an equals method for the class. The
witch considers two Child objects to be equal if their heights differ
by no more than 2 cm and their masses differ by no more than 0.5
kg.
- Write a definition of an equals method for the Fraction class. Your
method should return true if and only if the Fraction objects being
compared represent equivalent fractions.
| |