5.2 Parameters
The methods that we looked at in the previous section did exactly the same
thing every time that we called them. To make methods more flexible,
it is often desirable to be able to give them different values to start their
calculations. To see how to do this in Java, let us look at a simple example.
Example 1 The following method will print a line containing a given character (specified
by c) repeated a given number of times (specified by n). The method
will print a blank line if the value of n is less than one.
public static void printRow (char c, int n)
{
for (int i = 1; i <= n; i++)
System.out.print(c);
System.out.println();
}
|
|
In the definition of the method printRow in Example 1, the specification
(char c, int n) is called a parameter list. It serves as a declaration of
the variables c and n as the parameters of the method.
To use this method to write a line containing ten asterisks, we could
write the call in the form printRow('*' ,10); The values '*' and 10 are
called the arguments of the method.1
When a method like printRow is called, the values of the arguments are
automatically assigned to the method's parameters. The order in which the
parameters are written determines which argument gets assigned to which
parameter. We refer to this automatic transfer of values from arguments
to parameters as a passing of values.
Arguments are not restricted to being constants as we have shown so
far. They can be expressions or variables.
Example 2 Consider the statements
int m = 6;
printRow((char)('A'+3),m+2);
The call to printRow would cause the following sequence of actions to take
place.
- Using the value of the variable m at the point of the call, the arguments
of printRow would be evaluated.
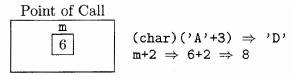
- These values would be automatically assigned to the parameters c
and n in the method printRow.
- Using these parameter values, the method would then be executed,
producing the output line
- Control would then be passed back to the statement following the
call to printRow.
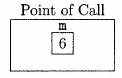
|
|
Be sure that you understand the process that Java uses in calls involving
parameters - an argument is evaluated and then this value is
automatically assigned to the corresponding parameter. This process is
known as call by value. Other programming languages use other parameter
passing mechanisms but Java uses call by value exclusively. One of the
consequences of this is that the communication between arguments and
parameters is in one direction only - from the arguments to the parameters.
The parameter is given a copy of the value of the argument, not the
argument itself.
Example 3 Consider the following method outline. (The dots indicate omitted material.)
public static void sample (int n)
{
...
n++;
...
}
Suppose now that we were to call this method by writing
int n = 3;
sample(n);
This would produce the following sequence of events.
- The value of the argument of sample is evaluated.
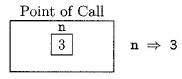
- This value is then assigned to the parameter n in the method sample.
The parameter variable n is completely distinct from the other variable
n.
- The method sample is then executed, changing the value of the parameter
n to 4.
- Once execution of the method has concluded, control returns to the
statement after the call. The value of n here is unchanged.
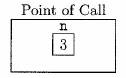
|
|
Since argument values are being assigned to parameters, the types of
arguments and parameters must be appropriate. Usually, this means that
arguments and parameters are of the same type but this is not necessarily
the case. Any matching in which an assignment of the argument type can
be made to the parameter type is acceptable.
Example 4 Consider the following method heading:
public static void doThis (int n, double x)
The arguments for this method would normally be an int followed by a
double but it would also be valid to call the method with a char and a
float or a byte and an int to name only some of the possibilities.
|
|
Exercise 5.2
- A student wrote the following method to exchange the values of two
int variables.
public static void swap (int m, int n)
{
int temp = m;
m = n;
n = temp;
}
He then tested the method with the following fragment:
i = 7;
j = 3;
swap(i,j) ;
System.out.println("i = " + i + " and j = " + j);
What did the fragment print? Explain.
- For the method doThis whose header was given in Example 4, state
which of the following calls are invalid. Justify your answer in each
case.
a. doThis(0.5,2); | b. doThis(3,4); |
c. doThis(2.0,1.5); | d. doThis(-2,8.0); |
e. doThis('x',5); | f. doThis(3L,4); |
- Complete the definition of the method printRect so that it
prints a filled rectangular pattern, using the character c, that is
width characters wide and height characters high.
public static void printRect(char c, int width, int height)
- Modify your method so that it prints an open rectangular pattern with blanks in the interior (if there is one).
| |
__________________________
1In other texts you may see parameters called formal parameters and arguments called actual parameters. We prefer the shorter names.